Let’s build a SwiftUI app that displays the NASA photo of the day. Updates will be tiny and on YouTube and GitHub
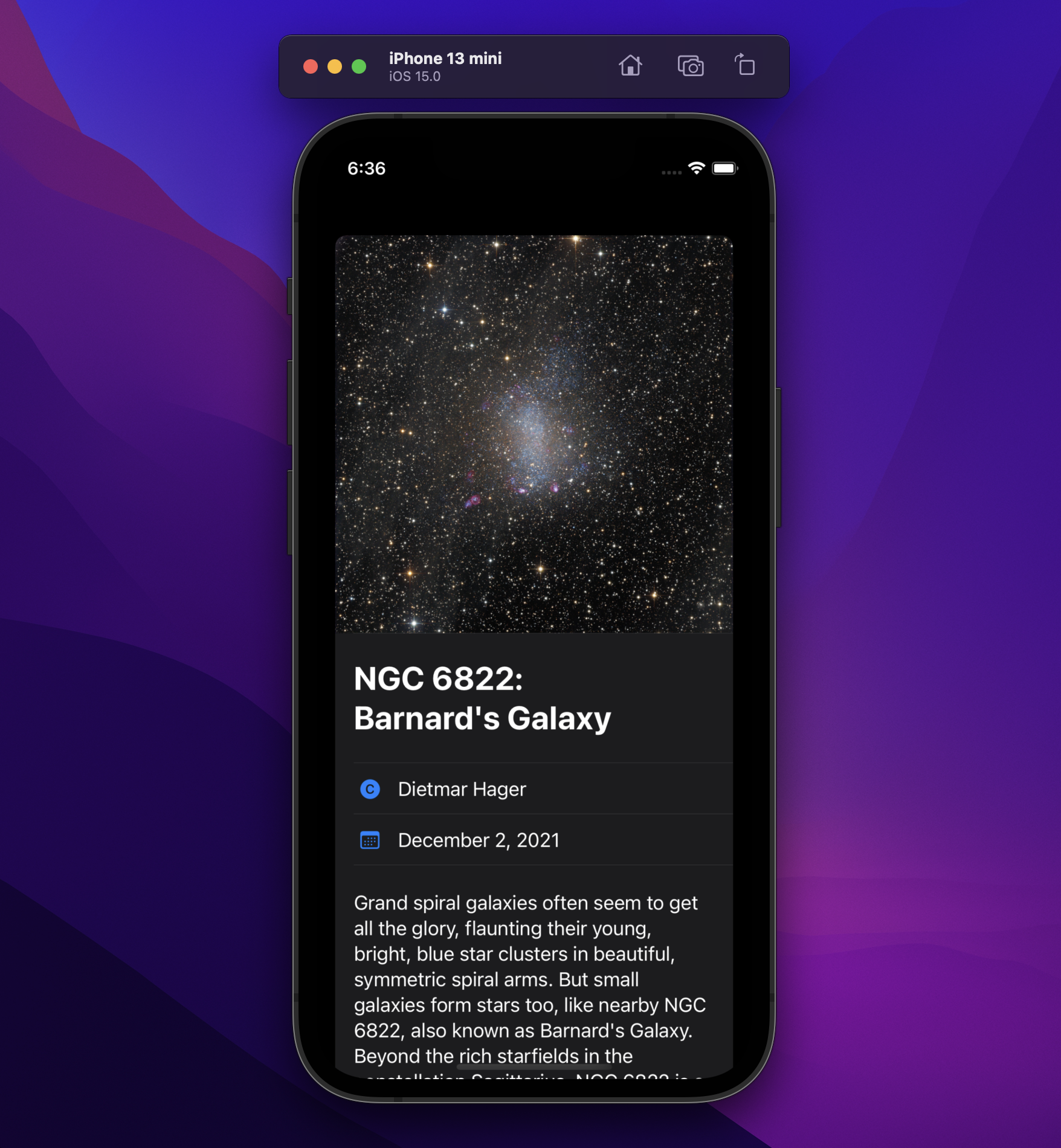
import SwiftUI
struct ContentView: View {
var body: some View {
List {
MyButton(title: "Button 1")
MyButton(title: "Button 2")
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
struct MyButton: View {
var title: String
@State private var showSheet = false
var body: some View {
Button(action: {
showSheet.toggle()
}, label: {
Text(title)
}).sheet(isPresented: $showSheet, content: {
Text("Sheet for \(title)")
})
}
}